Useful notifications from your home appliances using Node-RED
Some time ago I worked on a home project to get a notification when my washing machine had done its job based on monitoring its power consumption. There was a good reason for that, the machine was outside the house and I had already forgotten about the laundry several times. And when that happens your only option is to wash it again, because it really smells musty…
Monitoring your appliances
Use ESPurna :)
OK, there are different ways to get the info about power consumption. But since we want to be able to process the data ourselves most commercial products won't be suitable unless we modify it.
Alternatively, those that use radio communication to send data from the meter to the base station might be suitable for a man-in-the-middle hack. For instance, if you own an Efergy power meter you must know you can sniff the data it sends using a simple RTL-SDR dongle.
But for most cases, your best chance is to get your hands on a commercial product with an ESP8266 chip in it and change the firmware to suit your needs. You can write your own or use an existing firmware like ESPurna, that already supports a bunch of power metering smart switches.
What info do you need?
The idea is to report (via MQTT) power data from each individual appliance very minute. You can then use Node-RED along with InfluxDB and Grafana (or Graphite) to receive, persist and graph your data like in the image below.

There is a lot of useful information you can get just by graphing this data: device power footprint, device health, power consumption habits, duty cycles (for the fridge, for instance), max minute power (a key factor in your electricity bill) and, of course, foresee your next bill.
The info you will be getting has two main limitations: sensor precision (and error) and data granularity. Your sensors (clamps, power monitoring chips,…) will have a precision of maybe 5, 10 or 20W. And, even thou you are maybe reading them every few seconds you are probably averaging to get minute readings.
With this info, you will not be able to “see” an LED light turning on and off and you will probably get a baseline of a few tens of watts that you won't know where they exactly come from. But for some “big” appliances like your washer machine, your dryer, the oven, the dishwasher and such, you will get a pretty accurate footprint since their duty cycles usually last for 30 minutes, 60 minutes or even more.
One thing you can do is to monitor their power consumption to know when they are done (my washer machine example before). But since you have some significant data you can think on adding a bit more info to that “your laundry is done” message.
Analysing the data with Node-RED
The simplest analysis you can do on the power data is to know if the appliance is using any power at all. A value over 0W would then trigger a counter that will be aggregating energy (power times time) until the power goes back to 0W.
Problem is that some appliances might have duty cycles with periods of inactivity and you might also face the problem of having spurious or non-zero baseline power values (those damned LED pilots!). So you might want to set the power threshold to something different than 0 and maybe also set a “grace time” before triggering the done event.
I've been doing this already using a function node in Node-RED for some time now. But now that I wanted to add more info and connect more appliances to the flow I decided to put all the logic into a proper Node-RED node.
node-red-contrib-power-monitor
The node-red-contrib-power-monitor node is released under the Apache 2.0 license and can be installed from the Node-RED Manage Palette, using npm or checked out from my node-red-contrib-power-monitor repository on GitHub.
The node (node-red-contrib-power-monitor) accepts a real number as an input payload (in Watts) and does the analysis of the info based on 3 settings: the power threshold, a start grace period and a stop grace period.
The node installation is very simple and it does not have any external dependency. You can install it directly from the Manage Palete in you Node-RED UI or using npm from your node-red folder (typically ~/.node-red):
npm i node-red-contrib-power-monitor
Using it
The node configuration allows you to make it match the appliance power footprint with simple settings:
- Name: Name of the appliance. Will be attached to the output object.
- Power threshold: Value (in watts) to tell whether the appliance is running or not, an ideal value would be 0 (0W if not running).
- Start after: Number of messages with readings over the threshold to trigger a start event.
- Stop after: Number of messages with readings below the threshold to trigger a stop event.
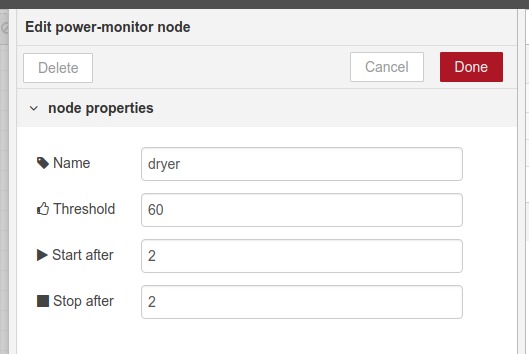
Examples
My washer machine below shows a clear and easy to analyze pattern. The power baseline is noiseless (I use a Sonoff POW R2) and the footprint does not show any 0W valley in between. Setting the default values for the node configuration (0W threshold and 1 for start and stop after counts) would work just fine.
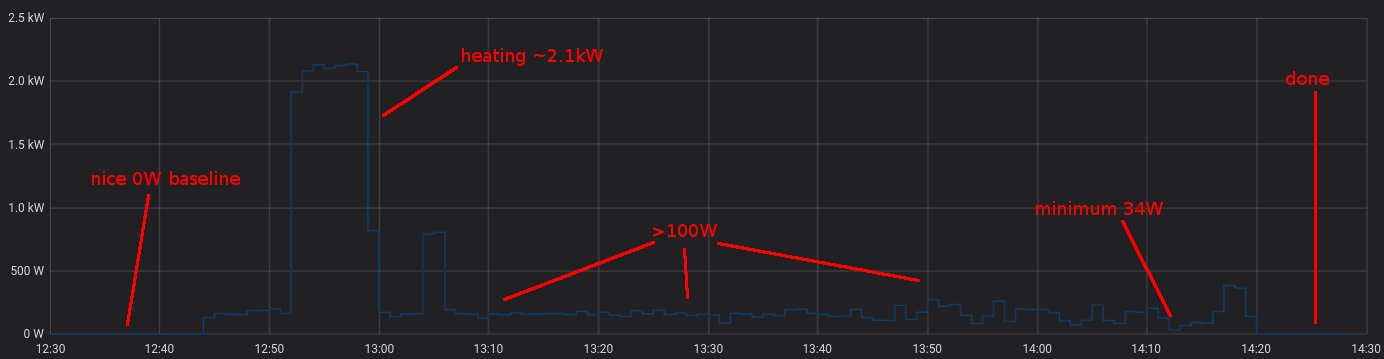
The dryer is more tricky, but still easy. As you can see the base line is not that clear (I'm using a Sonoff POW and it reads noise of up to 5W). But the activity power value is very obvious and over 500W constantly. Once it's done it enters a maintenance cycle to prevent wrinkles that lasts for an hour but the drying cycle is already finished. I'm using a threshold of 100W and leave the start and stop after counts to 1.
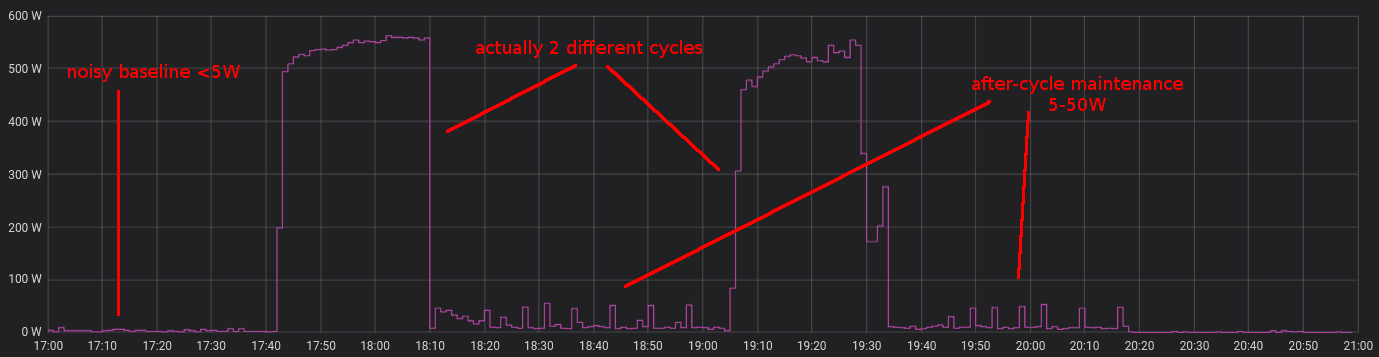
Finally, the dishwasher shows a more complex pattern with 3 different heating periods with low power activity in the middle raging from around 8W to more than 50W. In the end, there is a standby period with readings of around 3W. I'm using a custom board based on the HLW8012 power monitoring chip here, the same as in the POW but the baseline is pretty clean and reads 0W. I use a 5W threshold here but combined with a value for the start and stop counts of 5 to prevent false positives.
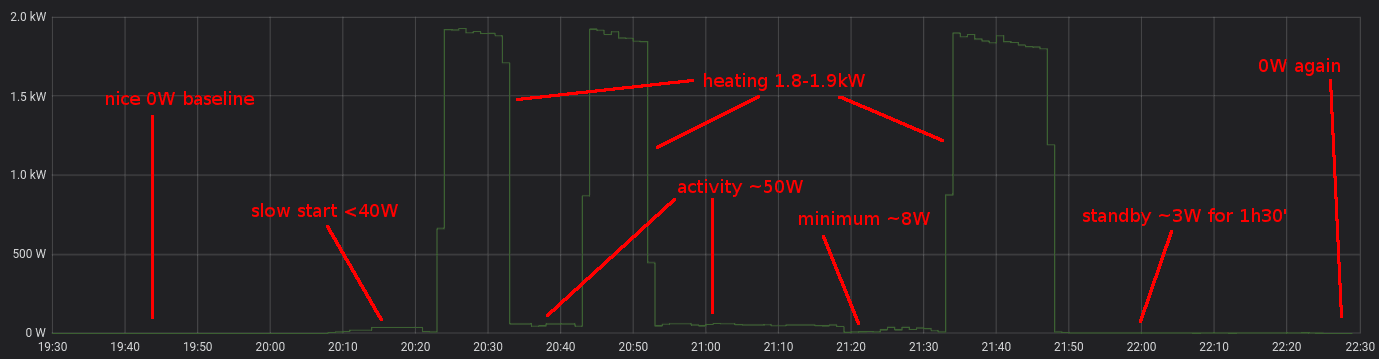
Output
Each node will provide real-time info about the running time and energy consumption so far for that given cycle.
The output is split into two, a connector for the start event and another one for the stop event. The start event is just that, a JSON payload with an event type “start” and the name of the appliance. The stop event adds a couple of very useful values: the total time the appliance has been running in seconds and the total energy in kWh.
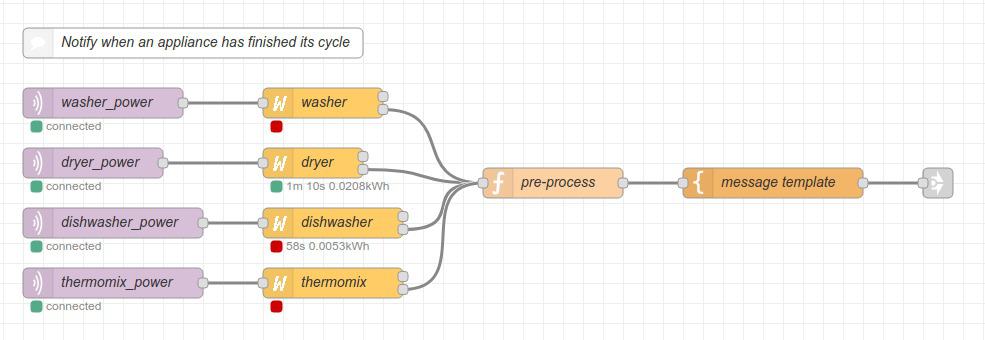
You might want to pre-process this info before feeding it to a template node to build a message. In the example above the “pre-process” function node calculates the time in minutes and the cost, assuming a flat rate, but it since you know the current time and the total running time you can make a fair estimate in case you have some kind of time discrimination.
Pre-process code:
msg.payload.time = Math.round(msg.payload.time / 60);
msg.payload.cost = Math.round(msg.payload.energy * 16.66) / 100;
return msg;
Message template (mustache syntax):
The {{ payload.name }} has finished.
Total time: {{ payload.time }} minutes.
Total energy: {{ payload.energy }} kWh.
Cost: {{ payload.cost }} €
Nice notifications using Telegram
Now that we have all the info it's time to set up nice notifications. A while ago Germán Martín published a screenshot of his phone receiving a notification from his @iotfridgesaver project. He was also using ESPuna and Node-RED and the results where superb. I just had to copy him :)
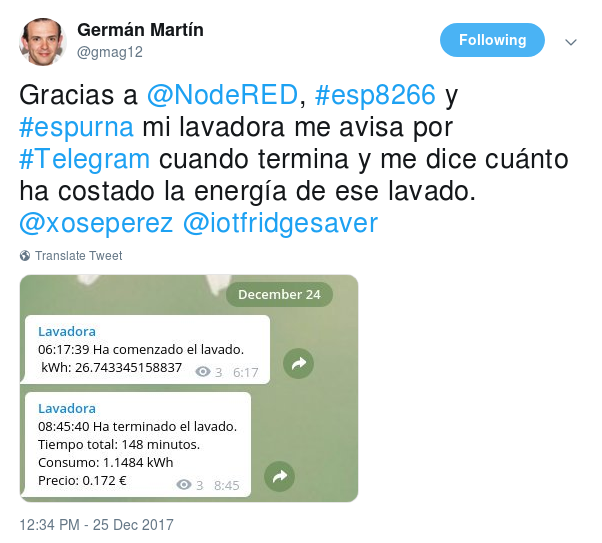
Creating a Telegram bot and start sending messages from Node-RED is really easy. You can find a lot of resources on the Internet so I won't go into the details. Just a small script of what you have to do:
-
Open a chat with @BotFather he-she-it will guide you through the process of creating a bot.
-
Install the node-red-contrib-telegrambot node in your Node-RED
-
Configure the token of the node to that provided by the @BotFather
-
You will also need a chatId where the bot has to send the messages. The easiest way is to use a bot like @my_id_bot. If you open a chat with it it will tell you your chatId so your bot will send you direct messages. If you invite @my_id_bot to a group it will tell you the group ID, very useful if you want to share notifications with someone else.
The node-red-contrib-telegrambot accepts a JSON payload with the info to send. You can use this template to build it from the previous payload:
{
"chatId": 224857347,
"type": "message",
"content": "{{ payload }}"
}
You can hardcode the chatId in the node or pass it as a parameter like in the example above. The result will look like this:
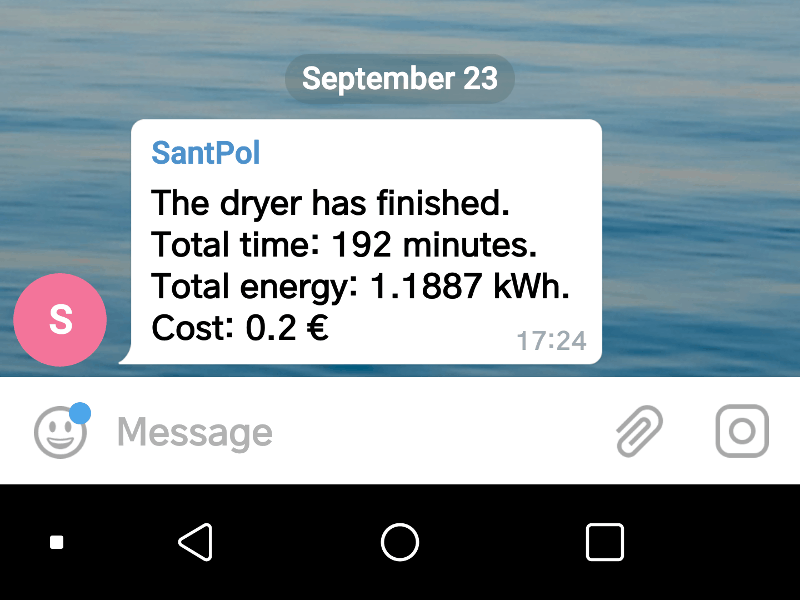
Cool?
"Useful notifications from your home appliances using Node-RED" was first posted on 24 September 2018 by Xose Pérez on tinkerman.cat under Code, Projects and tagged bot, botfather, efergy, esp8266, espurna, github, grafana, influxdb, node-red, node-red-contrib-power-monitor, notifications, power, rtl-sdr, telegram, threshold.