Playing slow catch with the Bean
A while ago I wrote about how to use PlatformIO with PunchThrough Lightblue Bean in a post here on how to use the new Bean Loader CLI from PlatformIO. Of course the reason for that was not merely being able to do it, but having a agile development environment to do something useful with them.
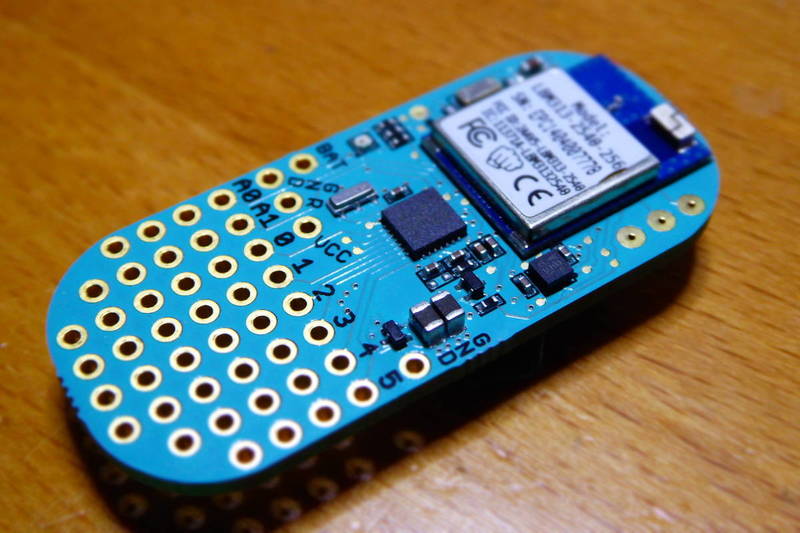
I've been looking for a paper I had read a few weeks before I started playing with the Beans. But I have not been able to find it. A few weeks before I had read the doctorate thesis [PDF] “Designing wearable and playful accessories to encourage free-play amongst school aged children: conception, participatory design and evaluation” by Andrea Rosales where she wrote about using sensors and controllers to create simple but fun games for the kids. One of the projects described in the paper was a “play catch” game where the kids had to chase each other trying not to trigger and alarm they had on a bracelet with an accelerometer. If they ran too fast or move too sharply an LED would flash and they had to stop until it went off.
Hardware
The Punchthrough Ligthblue Bean is a small board with a bluetooth enabled microcontroller, a 3-axis accelerometer and an RGB LED. It also has a small prototyping board and can be powered by a CR2032 coin cell. It's actually a nice piece of hardware although it doesn't come cheap.
You can code it using Arduino framework adding a few custom libraries to manager the bluetooth communication, the accelerometer and the RGB LED. With a few more components you can create really fun wearables.
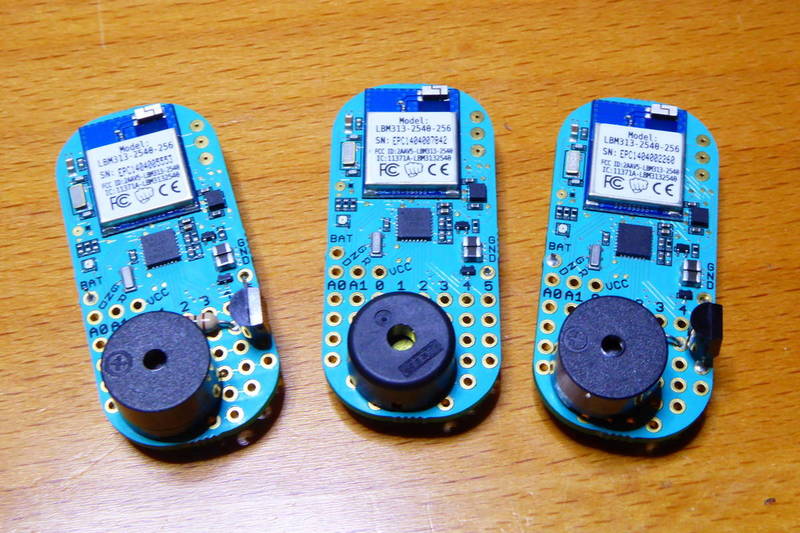
For this project the BOM is:
- A Punchthrough Lightblue Bean
- A CR2032 coin cell
- A buzzer
- A PN2222A NPN transistor
- A 1K resistor
The schematic is very simple. The transistor switches the power to the buzzer and you drive the transistor gate using a digital IO pin with the resistor in series. The 3V of the coin cell are enough to make the buzzer sound but you will certainly not have a loud beep here so the device will work better on quiet environments: indoors or noise-less outdoors.
Code
The code is really straight forward so I will copy it here and comment a few lines of it.
#define BUZZER_PIN 0
#define ACCELERATION_RANGE 2
#define ACCELERATION_BASE (512/ACCELERATION_RANGE)
#define SENSITIVITY 0.15
#define STOP_TONE 100
#define STOP_TONE_DURATION 1000
#define STOP_DURATION 2000
#define RESUME_TONE 400
#define RESUME_TONE_DURATION 250
#define CHECK_DELAY 50
void beep(int note, int duration) {
if (note==0) {
Bean.sleep(duration);
} else {
tone(BUZZER_PIN, note, duration);
delay(duration);
noTone(BUZZER_PIN);
}
}
float getAccelerationModule() {
float x = (float) Bean.getAccelerationX();
float y = (float) Bean.getAccelerationY();
float z = (float) Bean.getAccelerationZ();
float g = sqrt(x*x+y*y+z*z) / ACCELERATION_BASE;
return g;
}
void setup() {
pinMode(BUZZER_PIN, OUTPUT);
uint8_t mode = Bean.getAccelerometerPowerMode();
if (mode != VALUE_LOW_POWER_10MS) {
Bean.setAccelerometerPowerMode(VALUE_LOW_POWER_10MS);
}
Bean.setAccelerationRange(ACCELERATION_RANGE);
Bean.setLed(0, 0, 0);
}
void loop() {
float g = getAccelerationModule();
if (abs(g-1) > SENSITIVITY) {
Bean.setLed(255, 0, 0);
beep(STOP_TONE, STOP_TONE_DURATION);
Bean.sleep(STOP_DURATION);
beep(RESUME_TONE, RESUME_TONE_DURATION);
Bean.setLed(0, 0, 0);
}
Bean.sleep(CHECK_DELAY);
}
As you can see the setup just configures the buzzer pin and the accelerometer. The main loop checks whether the acceleration module is greater than 1.15 (that's 1.15g) and triggers the LED and the buzzer sequence to notify the user she has gone too fast, forcing her to stop until the release beep sounds 2 seconds after.
Playing
My daughters had a good time playing slow catch with their Bean powered bracelets. It was actually funny to see how they moved in a very awkward way trying not to trigger the buzzer. They even invented new games like being the first to touch a tree branch with the hand they were wearing the sensor (not fair since one it taller than the other) or trying to grab an orange from the table. Most of the times they both failed to do it without triggering the sound as they burst out in laughs.
Improvements
The buzzer is really too low to be heard outdoors unless there is some silence, and that's something that simply does not happen when kids are involved. I might try to use a 12V 23A battery to power a 12V buzzer and a step-down for the microcontroller. It will certainly add some cost to the project but it might do the difference when playing outdoors.
"Playing slow catch with the Bean" was first posted on 02 April 2017 by Xose Pérez on tinkerman.cat under Projects and tagged bean, game, lightblue bean, platformio, punchthrough.